This is a fully functional license system for C# applications, designed to work with a web-based backend. It ensures software security by binding licenses to specific hardware and logging all authentication attempts. While a new version is in progress, this legacy version is only receiving critical fixes.
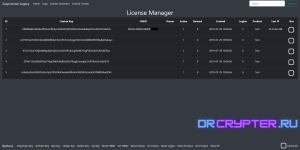
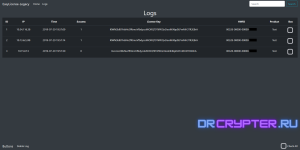
🌟 Features:
✅ License key binding – Each key is locked to the first-used PC (HWID-based).
✅ Security logging – Logs IP and HWID for every request, helping detect brute-force attempts.
✅ Built-in key generator – Generates 64-character alphanumeric license keys.
✅ Admin panel insights – View the last IP of each license key.
✅ Full license control – Reset, set HWID, deactivate keys, etc.
✅ Ban & remove – Banned users' software is auto-deleted, and their PC shuts down. 😲
🔍 Admin Panel Screenshots:
🚀 Installation Guide:
1️⃣ Create a MySQL database and import the provided panel.sql file.
2️⃣ Edit the configuration files (admin/config/db.php & login/db.php) with your database credentials.
3️⃣ Ensure the admin panel is working by generating some test keys.
4️⃣ Integrate C# files into your project and configure the URL & product name (see example in C#/EasyLicenseExample).
✅ If all steps are completed correctly, your license system should be up and running! 🎉
🎭 Code in c# sample :
📌 Example C# Code for License Authentication:
string key = "PUIuxZktNjvrZD7KQutLwa8RRZVJk1aXtadzI1Bn4K0dAGtyJoY8r4UEqqSBY36y";
string url = " ";
string productName = "Demo";
var license = new EasyLicense.License.Authorize();
license.ServerAuthUrl = url;
license.ProductName = productName;
bool success = license.Auth(key);
if (!success)
{
System.Console.WriteLine("Auth fail!");
System.Console.WriteLine("Reason: " + license.ResponseStatus);
}
else
{
System.Console.WriteLine("Success!");
}
🛠 How It Works:
1. The license key is stored in key.
2. The server URL is set in url.
3. The product name is assigned to productName.
4. The license.Auth(key) function checks if the key is valid.
5. If authentication fails, the system prints the failure reason.
6. If successful, it confirms authentication with a success message. ✅
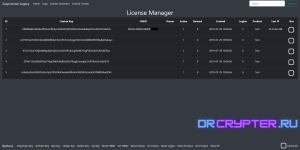
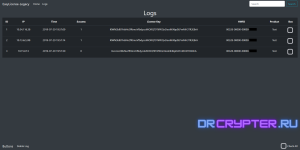
🌟 Features:
✅ License key binding – Each key is locked to the first-used PC (HWID-based).
✅ Security logging – Logs IP and HWID for every request, helping detect brute-force attempts.
✅ Built-in key generator – Generates 64-character alphanumeric license keys.
✅ Admin panel insights – View the last IP of each license key.
✅ Full license control – Reset, set HWID, deactivate keys, etc.
✅ Ban & remove – Banned users' software is auto-deleted, and their PC shuts down. 😲
🔍 Admin Panel Screenshots:
🚀 Installation Guide:
1️⃣ Create a MySQL database and import the provided panel.sql file.
2️⃣ Edit the configuration files (admin/config/db.php & login/db.php) with your database credentials.
3️⃣ Ensure the admin panel is working by generating some test keys.
4️⃣ Integrate C# files into your project and configure the URL & product name (see example in C#/EasyLicenseExample).
✅ If all steps are completed correctly, your license system should be up and running! 🎉
🎭 Code in c# sample :
📌 Example C# Code for License Authentication:
string key = "PUIuxZktNjvrZD7KQutLwa8RRZVJk1aXtadzI1Bn4K0dAGtyJoY8r4UEqqSBY36y";
string url = " ";
string productName = "Demo";
var license = new EasyLicense.License.Authorize();
license.ServerAuthUrl = url;
license.ProductName = productName;
bool success = license.Auth(key);
if (!success)
{
System.Console.WriteLine("Auth fail!");
System.Console.WriteLine("Reason: " + license.ResponseStatus);
}
else
{
System.Console.WriteLine("Success!");
}
🛠 How It Works:
1. The license key is stored in key.
2. The server URL is set in url.
3. The product name is assigned to productName.
4. The license.Auth(key) function checks if the key is valid.
5. If authentication fails, the system prints the failure reason.
6. If successful, it confirms authentication with a success message. ✅